Shortest Path Algorithm in C Language (Floyd algorithm)
Higher cateogyr : [C Language] C Language Table of Contents
2. Shortest path algorithm implemented in C language
a. GitHub
1. (Reference) Various distance concepts
⑴ Distance function: Defines the distance
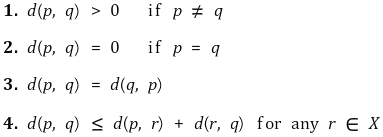
⑵ Type 1: L1 distance
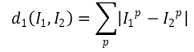
⑶ Type 2: L2 distance: Euclidean distance using the Pythagorean theorem (Standard)
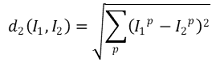
⑷ Type 3: p-norm

⑸ Type 4: dot product
⑹ Type 5: linkage metric: Defines the distance between clusters
⑺ Type 6: [KL distance](https://jb243.github.io/pages/2145#:~:text=%E2%91%A7-,%EC%83%81%EB%8C%80%20%EC%97%94%ED%8A%B8%EB%A1%9C%ED%94%BC,-(relative%20entropy%2C)(Kullback-Leibler divergence)
⑻ Type 7: Hamming distance: Measures the distance between data points by specifying binary values for each of them
⑼ Type 8: Mahalanobis distance: Measures the distribution of data
2. Shortest path algorithm implemented in C language
⑴ Problem situation: For example, the path from 0 to 5 requires a cost of 6. In other words, it requires moving a distance of 6.
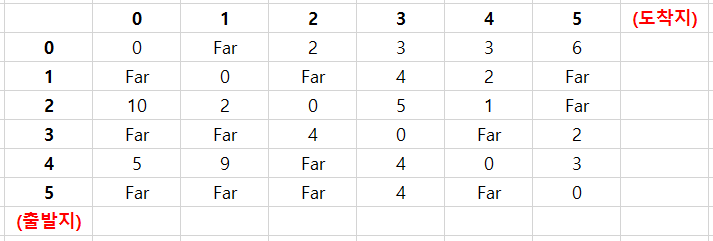
⑵ C language code
#include <stdio.h>
#include <stdlib.h>
#define Count_Vertice 6
#define Far_Distance 2000
int W[Count_Vertice][Count_Vertice] = {
// W[i][j] represents the direct distance from i to j, where a large value indicates that direct travel is not possible
{0, Far_Distance, 2, 3, 3, 6},
{Far_Distance, 0, Far_Distance, 4, 2, Far_Distance},
{10, 2, 0, 5, 1, Far_Distance},
{Far_Distance, Far_Distance, 4, 0, Far_Distance, 2},
{5, 9, Far_Distance, 4, 0, 3},
{Far_Distance, Far_Distance, Far_Distance, 4, Far_Distance, 0},
};
int D[Count_Vertice][Count_Vertice]; // D[i][j] stores the minimum distance from i to j
int P[Count_Vertice][Count_Vertice]; // P[i][j] stores the highest degree vertex traversed from i to j
void Floyd(){
int i, j, k;
for(i=0; i < Count_Vertice; i++) // Initialize arrays
for(j=0; j < Count_Vertice; j++){
P[i][j] = -1;
D[i][j] = W[i][j];
}
for(k=0; k < Count_Vertice; k++)
for(i=0; i < Count_Vertice; i++)
for(j=0; j < Count_Vertice; j++)
if(D[i][j] > D[i][k] + D[k][j]){
/* If D[i][j] becomes shorter by going through k */
D[i][j] = D[i][k] + D[k][j];
P[i][j] = k;
}
}
void Print_Path(int a, int b){ // Note that i and j in Print_Path[i][j] are not printed
if(P[a][b] != -1) { // P[a][b] = -1 "=" the shortest distance is from a directly to b
Print_Path(a, P[a][b]);
printf("%d ", P[a][b]);
Print_Path(P[a][b], b);
}
}
int main(int argc, char *argv[]){
Floyd();
int a, b;
printf("Enter the starting point and the destination. (0 ~ %d)\n", Count_Vertice - 1);
scanf("%d %d", &a, &b);
printf("Shortest distance: %d\n", D[a][b]);
printf("Shortest path: ");
printf("%d ", a); Print_Path(a, b);
if(D[a][b] != 0) printf("%d", b); // D[a][b] = 0
"=" a = b
return 0;
}
Input: December 13, 2013, 22:55